The MMA8451 is a low-power accelerometer with 14 bits of resolution, with the following features:
- Embedded functions with flexible user-programmable options, configurable to two interrupt pins
- Embedded interrupt functions for overall power savings relieving the host processor from continuously polling data
- Access to both low-pass filtered data as well as high-pass filtered data, which minimizes the data analysis required for jolt detection and faster transitions
- Inertial wake-up interrupt signals from any combination of the configurable embedded functions allowing the MMA8451Q to monitor events and remain in a low-power mode during periods of inactivity
Features
- 1.95 to 3.6-volt supply voltage
- 1.6 to 3.6-volt interface voltage
- ±2g/±4g/±8g dynamically selectable full-scale
- Output data rates (ODR) from 1.56 Hz to 800 Hz
- 99 μg/√Hz noise
- 14-bit and 8-bit digital output
- I²C digital output interface (operates to 2.25 MHz with 4.7 kΩ pull-up)
- Two programmable interrupt pins for seven interrupt sources
- Three embedded channels of motion detection
- Freefall or motion detection: one channel
- Pulse detection: one channel
- Jolt detection: one channel
- Orientation (portrait/landscape) detection with programmable hysteresis
- Automatic ODR change for auto-wake and return to sleep
- 32 sample FIFO
- High pass filter data available per sample and through the FIFO
- Self-test
Again due to the size and package of the module its easier to buy a module, here is one that I purchased. All you need to do is solder on the header and then connect using wires from your module to your Wemos Mini, wiring connections later
Connection
I used the following connection from the module above to my Wemos Mini
micro:bit Connection | Module Connection |
3v3 | VCC_IN |
Gnd | Gnd |
P20 | SDA |
P19 | SCL |
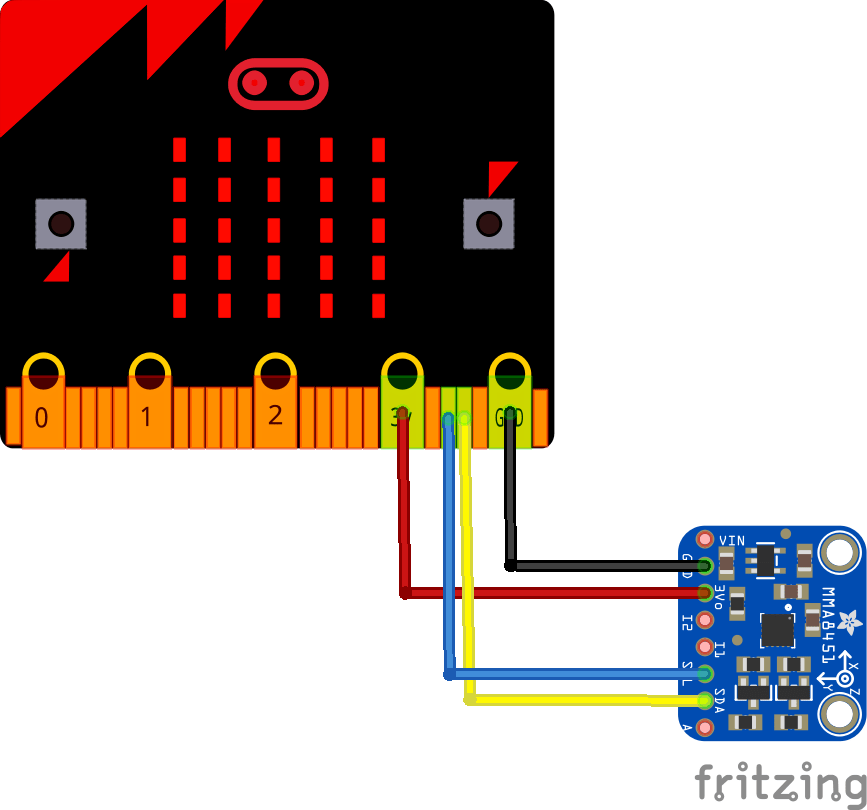
microbit and mma8451
Code
This requires the Adafruit library from https://github.com/adafruit/Adafruit_MMA8451_Library/archive/master.zip
I had to edit the Adafruit header file called Adafruit_MMA8451.h as my device was address 0x1c (28), I discovered this using an I2C scanner
#define MMA8451_DEFAULT_ADDRESS (0x1C)
[codesyntax lang=”cpp”]
#include <Wire.h> #include "Adafruit_MMA8451.h" #include <Adafruit_Sensor.h> Adafruit_MMA8451 mma = Adafruit_MMA8451(); void setup(void) { Serial.begin(9600); Serial.println("Adafruit MMA8451 test!"); if (! mma.begin()) { Serial.println("Couldnt start"); while (1); } Serial.println("MMA8451 found!"); mma.setRange(MMA8451_RANGE_2_G); Serial.print("Range = "); Serial.print(2 << mma.getRange()); Serial.println("G"); } void loop() { // Read the 'raw' data in 14-bit counts mma.read(); Serial.print("X:\t"); Serial.print(mma.x); Serial.print("\tY:\t"); Serial.print(mma.y); Serial.print("\tZ:\t"); Serial.print(mma.z); Serial.println(); /* Get a new sensor event */ sensors_event_t event; mma.getEvent(&event); /* Display the results (acceleration is measured in m/s^2) */ Serial.print("X: \t"); Serial.print(event.acceleration.x); Serial.print("\t"); Serial.print("Y: \t"); Serial.print(event.acceleration.y); Serial.print("\t"); Serial.print("Z: \t"); Serial.print(event.acceleration.z); Serial.print("\t"); Serial.println("m/s^2 "); /* Get the orientation of the sensor */ uint8_t o = mma.getOrientation(); switch (o) { case MMA8451_PL_PUF: Serial.println("Portrait Up Front"); break; case MMA8451_PL_PUB: Serial.println("Portrait Up Back"); break; case MMA8451_PL_PDF: Serial.println("Portrait Down Front"); break; case MMA8451_PL_PDB: Serial.println("Portrait Down Back"); break; case MMA8451_PL_LRF: Serial.println("Landscape Right Front"); break; case MMA8451_PL_LRB: Serial.println("Landscape Right Back"); break; case MMA8451_PL_LLF: Serial.println("Landscape Left Front"); break; case MMA8451_PL_LLB: Serial.println("Landscape Left Back"); break; } Serial.println(); delay(500); }
[/codesyntax]
Output
Open the serial monitor
X: 4686 Y: 2748 Z: 684
X: 10.80 Y: 6.11 Z: 0.21 m/s^2
Landscape Right Front
X: 1314 Y: -3198 Z: 2014
X: 2.01 Y: -7.75 Z: 5.52 m/s^2
Portrait Up Front
X: 2194 Y: 2762 Z: -2530
X: 6.57 Y: 7.33 Z: -2.77 m/s^2
Portrait Down Back
X: 2294 Y: 3182 Z: -392
X: 3.49 Y: 2.23 Z: 0.13 m/s^2
Landscape Right Back
X: 224 Y: 1324 Z: -2844
X: -2.46 Y: 7.03 Z: -9.81 m/s^2
Portrait Down Back
Links
Here is a link to the module
GY-45 MMA8451 Module Digital Triaxial Accelerometer High-precision Inclination Module